11.7: Fourier Transforms
- Page ID
- 84391
\( \newcommand{\vecs}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vecd}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash {#1}}} \)
\( \newcommand{\id}{\mathrm{id}}\) \( \newcommand{\Span}{\mathrm{span}}\)
( \newcommand{\kernel}{\mathrm{null}\,}\) \( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\) \( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\) \( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\inner}[2]{\langle #1, #2 \rangle}\)
\( \newcommand{\Span}{\mathrm{span}}\)
\( \newcommand{\id}{\mathrm{id}}\)
\( \newcommand{\Span}{\mathrm{span}}\)
\( \newcommand{\kernel}{\mathrm{null}\,}\)
\( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\)
\( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\)
\( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\inner}[2]{\langle #1, #2 \rangle}\)
\( \newcommand{\Span}{\mathrm{span}}\) \( \newcommand{\AA}{\unicode[.8,0]{x212B}}\)
\( \newcommand{\vectorA}[1]{\vec{#1}} % arrow\)
\( \newcommand{\vectorAt}[1]{\vec{\text{#1}}} % arrow\)
\( \newcommand{\vectorB}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vectorC}[1]{\textbf{#1}} \)
\( \newcommand{\vectorD}[1]{\overrightarrow{#1}} \)
\( \newcommand{\vectorDt}[1]{\overrightarrow{\text{#1}}} \)
\( \newcommand{\vectE}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash{\mathbf {#1}}}} \)
\( \newcommand{\vecs}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vecd}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash {#1}}} \)
\(\newcommand{\avec}{\mathbf a}\) \(\newcommand{\bvec}{\mathbf b}\) \(\newcommand{\cvec}{\mathbf c}\) \(\newcommand{\dvec}{\mathbf d}\) \(\newcommand{\dtil}{\widetilde{\mathbf d}}\) \(\newcommand{\evec}{\mathbf e}\) \(\newcommand{\fvec}{\mathbf f}\) \(\newcommand{\nvec}{\mathbf n}\) \(\newcommand{\pvec}{\mathbf p}\) \(\newcommand{\qvec}{\mathbf q}\) \(\newcommand{\svec}{\mathbf s}\) \(\newcommand{\tvec}{\mathbf t}\) \(\newcommand{\uvec}{\mathbf u}\) \(\newcommand{\vvec}{\mathbf v}\) \(\newcommand{\wvec}{\mathbf w}\) \(\newcommand{\xvec}{\mathbf x}\) \(\newcommand{\yvec}{\mathbf y}\) \(\newcommand{\zvec}{\mathbf z}\) \(\newcommand{\rvec}{\mathbf r}\) \(\newcommand{\mvec}{\mathbf m}\) \(\newcommand{\zerovec}{\mathbf 0}\) \(\newcommand{\onevec}{\mathbf 1}\) \(\newcommand{\real}{\mathbb R}\) \(\newcommand{\twovec}[2]{\left[\begin{array}{r}#1 \\ #2 \end{array}\right]}\) \(\newcommand{\ctwovec}[2]{\left[\begin{array}{c}#1 \\ #2 \end{array}\right]}\) \(\newcommand{\threevec}[3]{\left[\begin{array}{r}#1 \\ #2 \\ #3 \end{array}\right]}\) \(\newcommand{\cthreevec}[3]{\left[\begin{array}{c}#1 \\ #2 \\ #3 \end{array}\right]}\) \(\newcommand{\fourvec}[4]{\left[\begin{array}{r}#1 \\ #2 \\ #3 \\ #4 \end{array}\right]}\) \(\newcommand{\cfourvec}[4]{\left[\begin{array}{c}#1 \\ #2 \\ #3 \\ #4 \end{array}\right]}\) \(\newcommand{\fivevec}[5]{\left[\begin{array}{r}#1 \\ #2 \\ #3 \\ #4 \\ #5 \\ \end{array}\right]}\) \(\newcommand{\cfivevec}[5]{\left[\begin{array}{c}#1 \\ #2 \\ #3 \\ #4 \\ #5 \\ \end{array}\right]}\) \(\newcommand{\mattwo}[4]{\left[\begin{array}{rr}#1 \amp #2 \\ #3 \amp #4 \\ \end{array}\right]}\) \(\newcommand{\laspan}[1]{\text{Span}\{#1\}}\) \(\newcommand{\bcal}{\cal B}\) \(\newcommand{\ccal}{\cal C}\) \(\newcommand{\scal}{\cal S}\) \(\newcommand{\wcal}{\cal W}\) \(\newcommand{\ecal}{\cal E}\) \(\newcommand{\coords}[2]{\left\{#1\right\}_{#2}}\) \(\newcommand{\gray}[1]{\color{gray}{#1}}\) \(\newcommand{\lgray}[1]{\color{lightgray}{#1}}\) \(\newcommand{\rank}{\operatorname{rank}}\) \(\newcommand{\row}{\text{Row}}\) \(\newcommand{\col}{\text{Col}}\) \(\renewcommand{\row}{\text{Row}}\) \(\newcommand{\nul}{\text{Nul}}\) \(\newcommand{\var}{\text{Var}}\) \(\newcommand{\corr}{\text{corr}}\) \(\newcommand{\len}[1]{\left|#1\right|}\) \(\newcommand{\bbar}{\overline{\bvec}}\) \(\newcommand{\bhat}{\widehat{\bvec}}\) \(\newcommand{\bperp}{\bvec^\perp}\) \(\newcommand{\xhat}{\widehat{\xvec}}\) \(\newcommand{\vhat}{\widehat{\vvec}}\) \(\newcommand{\uhat}{\widehat{\uvec}}\) \(\newcommand{\what}{\widehat{\wvec}}\) \(\newcommand{\Sighat}{\widehat{\Sigma}}\) \(\newcommand{\lt}{<}\) \(\newcommand{\gt}{>}\) \(\newcommand{\amp}{&}\) \(\definecolor{fillinmathshade}{gray}{0.9}\)By Carey A. Smith
The code in this section is in file: FFT_demo_1.m
The Fourier Transform is defined as the integral of a signal multiplied by sine and cosine functions of frequencies which are multiples of a fundamental frequency.
A common use of FFT's is to find the frequency components of a signal.
% Computers compute the Fourier Transform of a signal vector using an algorithm called the "Fast Fourier Transform" or FFT.
MATLAB's function is fft(). The input is a vector y of signal values sampled at times specified by a vector t. For a signal vector, y, and a time vector, t, the syntax to properly scale the frequencies is:
y_FFT = (sqrt(t(end)))*fft(y);
%% This example shows the use of the Fourier Transform for spectral analysis.
clear all; close all; format compact; format short; clc;
% Create a time vector
nt = 256; % Vector length (A power of 2 is most efficient)
dt = 0.01; % (sec) Sampling interval
t = (0:1:(nt-1))*dt; % (sec) Time vector
%% Create a Signal vector
bias = 0; % Average value
f1 = 4.0 % (Hz) 1st frequency
a1 = 4.0 % Magnitude of the 1st frequency
f2 = 10.0 % (Hz) 2nd frequency
a2 = 2.0 % Magnitude of the 2nd frequency
y = bias + a1*sin(2*pi*f1*t) + a2*sin(2*pi*f2*t);
y_power = mean(y.^2) % power in the signal
%% Plot y vs. t
figure;
plot(t,y)
title('Original Signal vs. Time')
xlabel('Time (s)')
grid on;
%% Compute the Fourier Transform
y_FFT = (sqrt(t(end)))*fft(y); % Scale the FFT
f_max = (1/dt) % (Hz) Maximum frequency = Sample frequency
df = (1/dt)/nt % (Hz) Freq. interval
freqs = (0:1:(nt-1))*df; % Frequencies of the FFT
%% Plot the FFT of y
% The FFT result vector has complex values
figure;
plot(freqs,real(y_FFT),'b');
hold on;
plot(freqs,imag(y_FFT),'r');
legend('Real(y\_FFT)','Imag(y\_FFT)');
title('FFT(y))');
xlabel('Frequency (Hz)');
grid on;
%% Convert the complex Fourier Transform to magnitude squared.
y_FFT2 = (df/nt^2)*(y_FFT.*conj(y_FFT)); % (df/nt^2)is a scaled factor
y_FFT2_power = sum(y_FFT2)
% 10.02
figure;
plot(freqs,y_FFT2);
title('FFT2 Power)');
xlabel('Frequency (Hz)');
grid on;
%% The Nyquist–Shannon sampling theorem says
% you can only measure frequencies up to 1/2 the sampling frequency.
f_Nyquist = f_max/2
% You can see that the power plot is symmetric about the center frequency.
% The frequencies > f_Nyquist are equivalent to negative frequncies.
% We double the lower frequncies (except for 0 Hz)
% and delete the high frequencies.
y_FFT3 = [y_FFT2(1), 2*y_FFT2(2:(nt/2)), y_FFT2(nt/2+1)];
y_FFT3_power = sum(y_FFT3)
% 10.02
freqs3 = freqs(1:(nt/2+1));
figure;
plot(freqs3,y_FFT3);
title('y\_FFT3 Power Spectrum');
xlabel('Frequency (Hz)');
grid on;

Figure \(\PageIndex{1}\): Signal vs. time


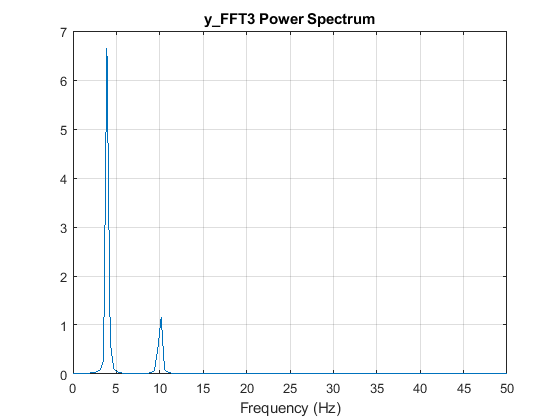
.
Modify the code in the above example as follows.
Change the 2 frequencies to 6 Hz and 16 Hz.
Call this signal y0. (This is without noise)
Use randn() to add a noise vector to y0 with a mean of 0 and a standard deviation of 4.
y = y0 + 4*randn(size(y));
This adds noise of all frequencies. Such noise is called “white noise”.
The Power Spectrum should be strong at the 2 main frequencies. A small amount of random power should be at all the other frequencies.
- Answer
-
Add texts here. Do not delete this text first.
Using your code from the previous exercise (Spectral Analysis 2), increase the noise to a point where the noise makes it somewhat hard to see the periodic part of signal in the plot of y vs. t.
Describe what the Fourier Transform plot shows.
Are the frequencies of the 2 sine waves identifiable?
- Answer
-
Add texts here. Do not delete this text first.