4.2: Activity 2 - Sorting Algorithm
- Page ID
- 49947
Introduction
This activity involves studying the sorting process and how to write algorithms that can order particular items in a list to be in given order. The activity of sorting ensures items in a given list are arranged in a desired order, say ascending or descending. The section involves studying insertion sort, shell sort, and quicksort algorithms.
The sorting algorithm
A sorting algorithm is defined as an algorithm that puts elements of a list in a certain order. The most-used order is the alphabetical order, also known as the lexicographical order. Efficient sorting has the importance for optimizing the use of other algorithms (such as search and merge algorithms) which require input data to be in sorted lists. Examples of sorting algorithms include: insertion sort, shell sort and quick sort.
Insertion sort is the simplest method, and does not require any additional storage. Shell sort is a simple modification that improves performance significantly. Quicksort is the most efficient and popular method, and is the method of choice for large arrays.
Insertion Sort
Insertion sort is a simple sorting algorithm, and which is relatively efficient for small lists and mostly sorted lists. It can also be used as part of more sophisticated algorithms. It works by taking elements from the list one by one and inserting them in their correct position into a new sorted list. In an array structure, the new list and the remaining elements can share the array’s space, but insertion is expensive, requiring shifting all following elements over by one. The array is searched sequentially and unsorted items are moved and inserted into sorted sublist (in the same array). This algorithm is not suitable for large data sets as its average and worst case complexity are of Ο(n2) where n is no. of items.
Insertion sort compares the first two elements:
It finds that both 14 and 33 are already in ascending order. For now, 14 is in sorted sub-list.
Insertion sort moves ahead and compares 33 with 27.
It swaps 33 with 27. Also it checks with all the elements of sorted sublist. Here we see that sorted sub-list has only one element 14 and 27 is greater than 14. Hence sorted sub-list remain sorted after swapping.
By now we have 14 and 27 in the sorted sublist. Next it compares 33 with 10.
These values are not in sorted order.
So we swap them.
But swapping makes 27 and 10 unsorted.
Again we find 14 and 10 in unsorted order.
And we swap them. By the end of third iteration we have a sorted sublist of 4 items.
This process goes until all the unsorted values are covered in sorted sublist. And now we shall see some programming aspects of insertion sort.
Example of the insertion algorithm
The following are simple steps by which we can achieve insertion sort.
Step 1 − If it is the first element, it is already sorted. return 1;
Step 2 − Pick next element
Step 3 − Compare with all elements in the sorted sub-list
Step 4 − Shift all the elements in the sorted sub-list that is greater than the value to be sorted
Step 5 − Insert the value
Step 6 − Repeat until list is sorted
Shell Sort
Shell sort improves on the efficiency of insertion sort by quickly shifting values to their destination. It improves upon bubble sort and insertion sort by moving out of order elements more than one position at a time. One implementation can be described as arranging the data sequence in a two-dimensional array and then sorting the columns of the array using insertion sort.
Shell sort is a highly efficient sorting algorithm and is based on insertion sort algorithm. This algorithm avoids large shifts as in case of insertion sort if smaller value is very far right and has to move to far left. This algorithm uses insertion sort on widely spread elements first to sort them and then sorts the less widely spaced elements. This spacing is termed as interval. This algorithm is quite efficient for medium sized data sets as its average and worst case complexity are of O(n) where n is no. of items.
How shell sort works
Consider the example below to have an idea how shell sort works. We take the same array we have used in our previous examples. For our example and ease of understanding we take the interval of 4. And make a virtual sublist of all values located at the interval of 4 positions. Here these values are {35, 14}, {33, 19}, {42, 27} and {10, 14}.
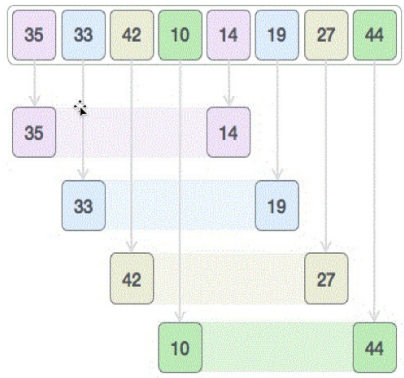
We compare values in each sub-list and swap them (if necessary) in the original array. After this step, the new array should look like this −
Then we take interval of 2 and this gap generates two sublists - {14, 27, 35, 42}, {19, 10, 33, 44}.
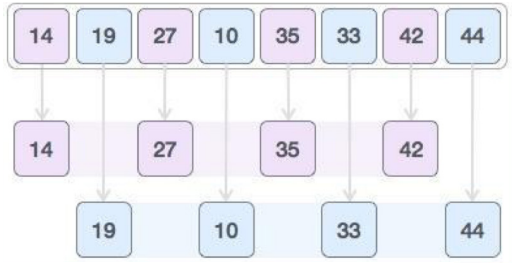
We compare and swap the values, if required, in the original array. After this step, this array should look like this −
And finally, we sort the rest of the array using interval of value 1. Shell sort uses insertion sort to sort the array. The step by step depiction is shown below −
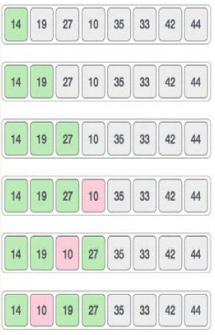
It can be seen that it required only four swaps to sort the rest of the array.
The following is the algorithm for shell sort.
Step 1 − Initialize the value of h
Step 2 − Divide the list into smaller sub-list of equal interval h
Step 3 − Sort these sub-lists using insertion sort
Step 4 − Repeat until complete list is sorted
Quicksort
It is one of the most popular sorting algorithms. It is significantly a better algorithm than the insertion sort.
Quick sort is a highly efficient sorting algorithm and is based on partitioning of array of data into smaller arrays. A large array is partitioned into two arrays, one of which holds values smaller than specified value say pivot based on which the partition is made, and another array holds values greater than pivot value.
The quick sort partitions an array and then calls itself recursively twice to sort the resulting two subarrays. This algorithm is quite efficient for large sized data sets as its average and worst case complexity are of O(nlogn) where n is no. of items.
Below is the longer version of an algorithm for a quicksort:
Step 1 − Choose the highest index value as pivot
Step 2 − Take two variables to point left and right of the list excluding pivot
Step 3 − Left points to the low index
Step 4 − Right points to the high
Step 5 − While value at left is less than pivot move right
Step 6 − While value at right is greater than pivot move left
Step 7 − If both step 5 and step 6 does not match swap left and right
Step 8 − If left ≥ right, the point where they met is new pivot
It can be summarized into the following fewer steps; using pivot algorithm recursively, we end-up with smaller possible partitions. Each partition is then processed for quick sort. We define recursive algorithm for quicksort as below −
Step 1 − Make the right-most index value pivot
Step 2 − Partition the array using pivot value
Step 3 − Quicksort left partition recursively
Step 4 − Quicksort right partition recursively
Conclusion
This activity introduced the learner to three different sort algorithms: the insertion sort, the shell sort and quicksort. A brief description of each was given.
- Describe briefly an insertion sorting algorithm.
- What is the difference between selection and insertion sorting?