3.2: Card toString
- Page ID
- 15285
When you create a new class, the first step is to declare the instance variables and write constructors. A good next step is to write toString
, which is useful for debugging and incremental development.
To display Card
objects in a way that humans can read easily, we need to map the integer codes onto words. A natural way to do that is with an array of String
s. We can create the array like this:
String[] suits = new String[4];
And then assign values to the elements:
suits[0] = "Clubs"; suits[1] = "Diamonds"; suits[2] = "Hearts"; suits[3] = "Spades";
Or we can create the array and initialize the elements at the same time, as we saw in Section 8.3:
String[] suits = {"Clubs", "Diamonds", "Hearts", "Spades"};
The state diagram in Figure 12.2.1 shows the result. Each element of the array is a reference to a String
.
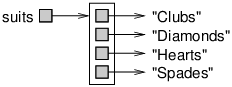
Now we need an array to decode the ranks:
String[] ranks = {null, "Ace", "2", "3", "4", "5", "6", "7", "8", "9", "10", "Jack", "Queen", "King"};
The zeroth element should never be used, because the only valid ranks are 1–13. We set it to null
to indicate an unused element.
Using these arrays, we can create a meaningful String
using suit
and rank
as indexes.
String s = ranks[card.rank] + " of " + suits[card.suit];
The expression suits[card.suit]
means “use the instance variable suit
from the object card
as an index into the array suits
.”
Now we can wrap all that in a toString
method.
public String toString() { String[] ranks = {null, "Ace", "2", "3", "4", "5", "6", "7", "8", "9", "10", "Jack", "Queen", "King"}; String[] suits = {"Clubs", "Diamonds", "Hearts", "Spades"}; String s = ranks[this.rank] + " of " + suits[this.suit]; return s; }
When we display a card, println
automatically calls toString
:
Card card = new Card(11, 1); System.out.println(card);
The output is Jack of Diamonds
.