8.4: Example One
- Page ID
- 54275
As previously described, writing or developing programs is easier when following a methodology. As the program becomes more complex, using a clear methodology is even more important. The main steps in the methodology are:
- Understand the Problem
- Create the Algorithm
- Implement the Program
- Test/Debug the Program
To help demonstrate this process in detail, these steps will be applied to a familiar problem as an example. The example problem is to calculate the solution of a quadratic equation in the form:
\[ ax^2 + bx + c = 0 \nonumber \]
Each of the steps, as applied to this problem, will be reviewed.
Understand the Problem
Before creating a solution, it is important to understand the problem. Ensuring a complete understanding of the problem can help reduce errors.
It is known that the solution to the quadratic equation is as follows:
\[ x = \frac{-b \pm \sqrt{(b^2 - 4ac)}}{2a} \nonumber \]
In the quadratic equation, the term \((b^2 - 4ac)\) is the discriminant of the equation. There are three possible results for the discriminant as described below:
- If \((b^2 - 4ac) > 0\) then there are two distinct real roots to the quadratic equation. These two solutions represent the two possible answers. If the equation solution is graphed, the curve representing the solution will cross the \(x\)-axis (i.e., representing \(x\)=0) in two locations.
- If \((b^2 - 4ac) = 0\) then there is a single, repeated root to the equation. If the equation solution is graphed, the curve representing the solution will cross the \(x\)-axis in one location.
- If \((b^2 - 4ac) < 0\) then there are two complex roots to the equation. If the equation solution is graphed, the curve representing the solution will not cross the \(x\)-axis and therefore there no real number solution. However, mathematically the square root of a negative value will provide a complex result. A complex number includes a real component and an imaginary component.
A correct solution must address each of these possibilities. For this problem, it is appropriate to use real values.
The relationship between the discriminant and the types of solutions (two different solutions, one repeated solution, or no real solutions) is summarized in the below table:
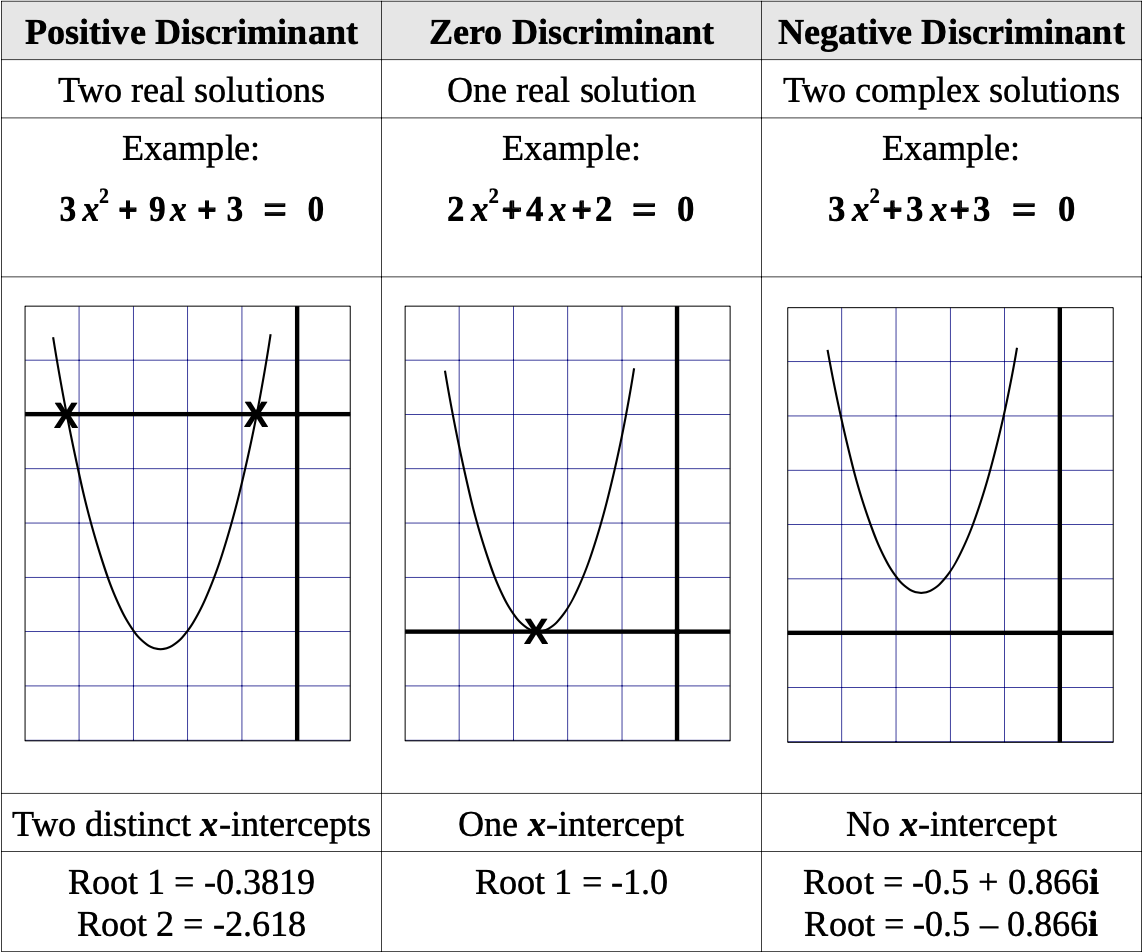
The examples provided above are included in the example solution in the following sections.
Create the Algorithm
The algorithm is the name for the ordered sequence of steps involved in solving the problem. The variables must be defined and an initial header displayed. For this problem, the \(a\), \(b\), and \(c\) values will need to be read from the user. Formalizing this, the following steps can be developed.
! declare variables ! reals -> a, b, c, discriminant, root1, root2 ! display initial header ! read the a, b, and c values
Then, the discriminant can be calculated.
Based on the discriminant value, the appropriate set of calculations can be performed.
! calculate the discriminant ! if discriminant is 0, ! calculate and display root ! if discriminant is >0, ! calculate and display root1 and root2 ! if discriminant is <0, ! calculate and display complex root1 and root2
For convenience, the steps are written as program comments.
Implement the Program
Based on the algorithm, the following program can be created.
program quadratic ! Quadratic equation solver program ! declare variables ! reals -> a, b, c, discriminant, root1, root2 implicit none real :: a, b, c real :: discriminant, root1, root2 ! display initial header write (*,*) "Quadratic Equation Solver Program" write (*,*) "Enter A, B, and C values" ! read the a, b, and c values read (*,*) a, b, c ! calculate the discriminant discriminant = b ** 2 – 4.0 * a * c ! if discriminant is 0, ! calculate and display root if ( discriminant == 0 ) then root1 = -b / (2.0 * a) write (*,*) "This equation has one root:" write (*,*) "root = ", root1 end if ! if discriminant is >0, ! calculate and display root1 and root2 if ( discriminant > 0 ) then root1 = (-b + sqrt(discriminant)) / (2.0 * a) root2 = (-b - sqrt(discriminant)) / (2.0 * a) write (*,*) "This equation has real roots:" write (*,*) "root 1 = ", root1 write (*,*) "root 2 = ", root2 end if ! if discriminant is <0, ! calculate and display complex root1 and root2 if ( discriminant < 0 ) then root1 = -b / (2.0 * a) root2 = sqrt(abs(discriminant)) / (2.0 * a) write (*,*) "This equation has complex roots:" write (*,*) "root 1 = ", root1, "+i", root2 write (*,*) "root 2 = ", root1, "-i", root2 end if end program quadratic
The indentation is not required, but does help make the program easier to read.
Test/Debug the Program
Once the program is written, testing should be performed to ensure that the program works. The testing will be based on the specific parameters of the program. In this example, each of the three possible values for the discriminant should be tested.
C:\mydir> quad Quadratic Equation Solver Program Enter A, B, and C values 2 4 2 This equation has one root: root = -1.0000000 C:\mydir> quad Quadratic Equation Solver Program Enter A, B, and C values 3 9 3 This equation has has real roots: root 1 = -0.38196602 root 2 = -2.6180339 C:\mydir> quad Quadratic Equation Solver Program Enter A, B, and C values 3 3 3 This equation has complex roots: root 1 = -0.50000000 +i 0.86602539 root 2 = -0.50000000 -i 0.86602539 C:\mydir>
Additionally, these results can be verified with a calculator.